Arrays
Arrays are a fundamental data structure in JavaScript used to store multiple values in a single variable. They can hold any type of data, including numbers, strings, objects, and even other arrays. Arrays are mutable, meaning you can change their contents by adding, removing, or updating elements.
Creating Arrays
const fruits = ['apple', 'banana', 'cherry'];
You can also use the Array constructor, but it's generally less common for simple arrays:
Accessing Elements
Array Properties and Methods:
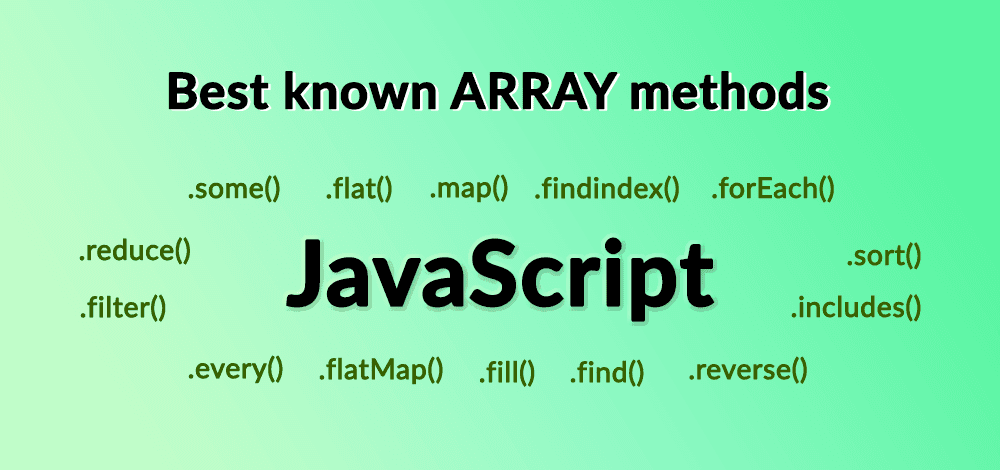
- push()
Adds one or more elements to the end of the array and returns the new length.- pop()
Removes the last element from the array and returns it.- shift()
Removes the first element from the array and returns it.- unshift()
Adds one or more elements to the beginning of the array and returns the new length.- slice()
Extracts a section of the array and returns a new array.- concat()
Merges two or more arrays and returns a new array.- indexOf()
Searches the array for a specific element and returns its index or -1 if not found.- forEach()
Executes a provided function once for each array element.- map()
Creates a new array with the results of calling a function on every element in the array.- filter()
Creates a new array with elements that pass a test implemented by the provided function.
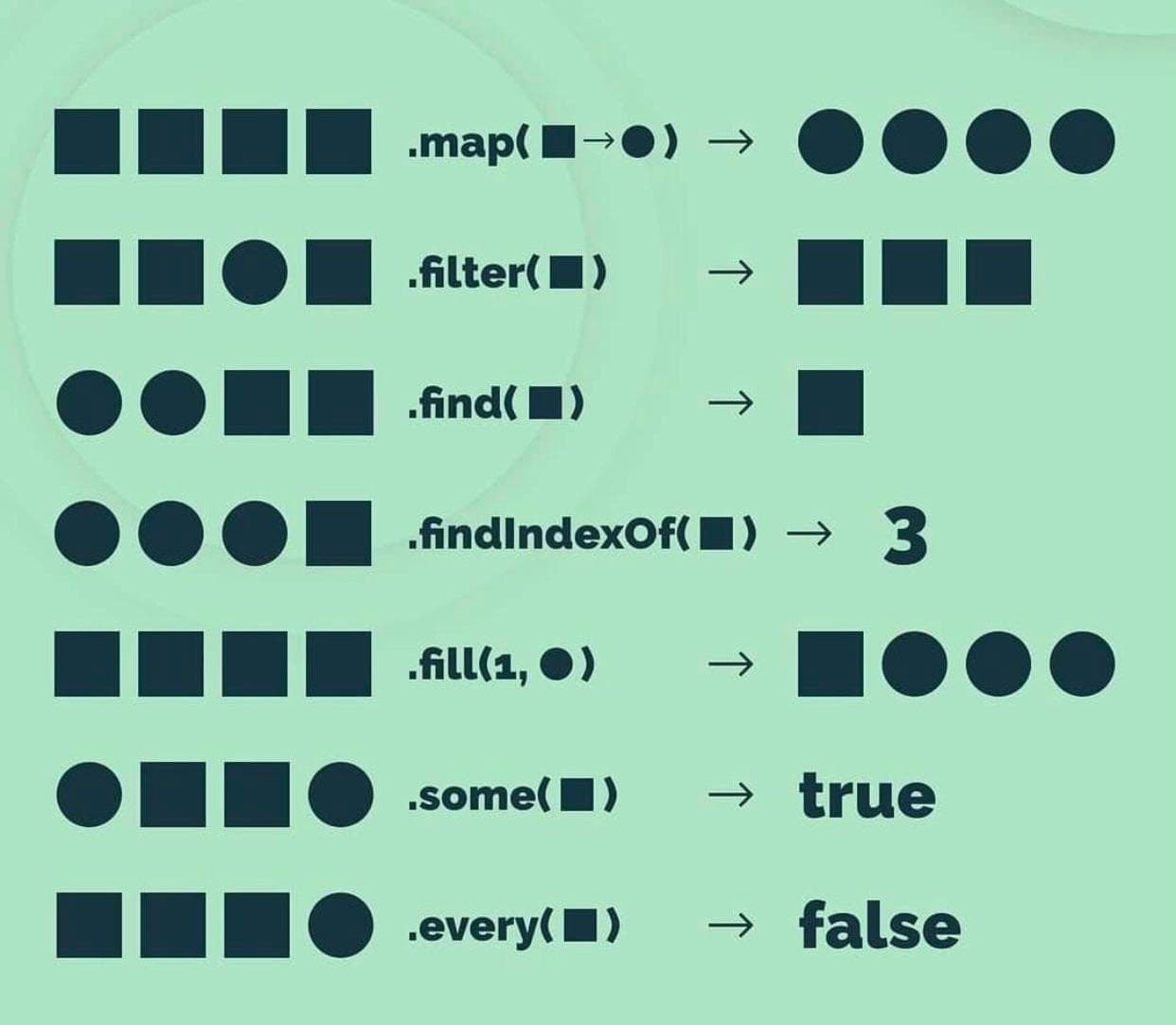