Control Structures in JavaScript
Control structures in JavaScript are fundamental elements that allow you to control the flow of execution in your program. They enable you to make decisions based on conditions, repeat code blocks a specific number of times, or iterate through collections of data. Here's a breakdown of the common control structures in JavaScript:
1. Conditional Statements:
if...else: This is the most basic conditional statement. It allows you to execute one block of code if a condition is true and another block if it's false. You can also add an optional else if clause to check for additional conditions.let num = 5;
if (num > 0) {
console.log(num + " is positive");
} else if (num < 0) {
console.log(num + " is negative");
} else {
console.log(num + " is zero");
}
switch:This structure is used for multi-way branching based on a single expression's value. It compares the expression's value with multiple case clauses and executes the associated code block if there's a match. A default case can be used to handle situations where no match is found.let day = "Tuesday";
switch (day) {
case "Monday":
console.log("Plan your week!");
break;
case "Tuesday":
case "Wednesday":
case "Thursday":
console.log("Work hard!");
break;
case "Friday":
console.log("Almost weekend!");
break;
default:
console.log("Enjoy the weekend!");
}
2. Looping Statements
forThis loop is used for a known number of iterations. It has three parts separated by semicolons: initialization, condition, and increment/decrement. The code block within the loop executes as long as the condition remains true, and the increment/decrement expression updates the loop counter after each iteration.for (let i = 0; i < 5; i++) {
console.log("Iteration", i + 1);
}
while:This loop continues executing its code block as long as a specified condition remains true. The condition is checked before each iteration, and the loop terminates when the condition becomes false.let count = 0;
while (count < 3) {
console.log("Count:", count);
count++;
}
do...while: Similar to while, this loop guarantees at least one execution of the code block even if the condition is initially false. The condition is checked after the code block executes, and the loop repeats as long as the condition is true.let count = 0;
do {
console.log("Count:", count);
count++;
} while (count < 0); // This will still log "Count: 0"
3,Jump Statements
break:This statement exits a loop prematurely, immediately transferring control to the code after the loop. It's typically used within conditional statements to break out of the loop when a certain condition is met.continue:This statement skips the remaining code block of the current iteration in a loop and jumps to the beginning of the next iteration. It's often used to conditionally skip specific iterations within a loop.By effectively using these control structures, you can write JavaScript programs that make decisions, repeat tasks, and process data efficiently.
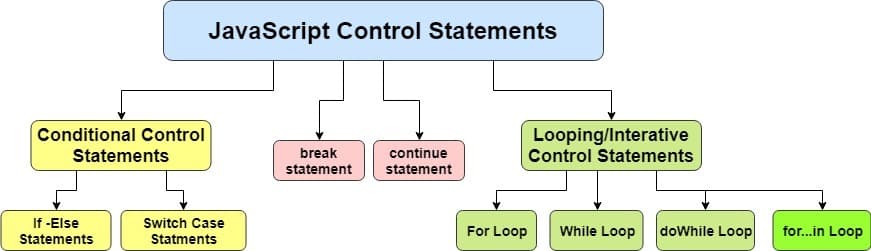